743-network-delay-time¶
Try it on leetcode
Description¶
You are given a network of n
nodes, labeled from 1
to n
. You are also given times
, a list of travel times as directed edges times[i] = (ui, vi, wi)
, where ui
is the source node, vi
is the target node, and wi
is the time it takes for a signal to travel from source to target.
We will send a signal from a given node k
. Return the time it takes for all the n
nodes to receive the signal. If it is impossible for all the n
nodes to receive the signal, return -1
.
Example 1:
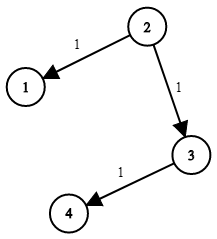
Input: times = [[2,1,1],[2,3,1],[3,4,1]], n = 4, k = 2 Output: 2
Example 2:
Input: times = [[1,2,1]], n = 2, k = 1 Output: 1
Example 3:
Input: times = [[1,2,1]], n = 2, k = 2 Output: -1
Constraints:
1 <= k <= n <= 100
1 <= times.length <= 6000
times[i].length == 3
1 <= ui, vi <= n
ui != vi
0 <= wi <= 100
- All the pairs
(ui, vi)
are unique. (i.e., no multiple edges.)
Solution(Python)¶
class Solution:
def networkDelayTime(self, times: List[List[int]], n: int, k: int) -> int:
return self.dijikstra(times, n, k)
# Time Complexity: O((N-1)!+E log E)
# Space COmplexity: O(N+E)
def dfs(self, times: List[List[int]], n: int, k: int) -> int:
adj = defaultdict(list)
for x, y, z in times:
adj[x].append((y, z))
times.sort(key=lambda time: time[2])
signalReceivedAt = [sys.maxsize] * (n)
def recur(node, currTime):
if currTime >= signalReceivedAt[node - 1]:
return
signalReceivedAt[node - 1] = currTime
for y, w in adj[node]:
recur(y, currTime + w)
recur(k, 0)
max_ = max(signalReceivedAt)
return max_ if max_ < sys.maxsize else -1
# Time Complexity: O(N*E)
# Space COmplexity: O(N+E)
def bfs(self, times: List[List[int]], n: int, k: int) -> int:
adj = defaultdict(list)
for x, y, z in times:
adj[x].append((y, z))
signalReceivedAt = [sys.maxsize] * (n)
q = deque([k])
signalReceivedAt[k - 1] = 0
while q:
node = q.popleft()
for y, w in adj[node]:
arrivalTime = signalReceivedAt[node - 1] + w
if arrivalTime < signalReceivedAt[y - 1]:
signalReceivedAt[y - 1] = arrivalTime
q.append(y)
max_ = max(signalReceivedAt)
return max_ if max_ < sys.maxsize else -1
# Time Complexity: O(N+ElogE)
# Space COmplexity: O(N+E)
def dijikstra(self, times: List[List[int]], n: int, k: int) -> int:
adj = defaultdict(list)
for x, y, z in times:
adj[x].append((y, z))
visited = set()
pq = [(0, k)]
heapq.heapify(pq)
maxcost = 0
while pq:
cost, node = heapq.heappop(pq)
if node in visited:
continue
if cost > maxcost:
maxcost = cost
visited.add(node)
for y, w in adj[node]:
newcost = cost + w
if y not in visited:
heapq.heappush(pq, (newcost, y))
return maxcost if n == len(visited) else -1