101-symmetric-tree¶
Try it on leetcode
Description¶
Given the root
of a binary tree, check whether it is a mirror of itself (i.e., symmetric around its center).
Example 1:
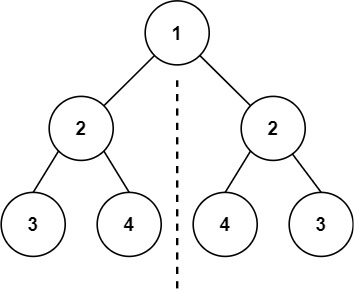
Input: root = [1,2,2,3,4,4,3] Output: true
Example 2:
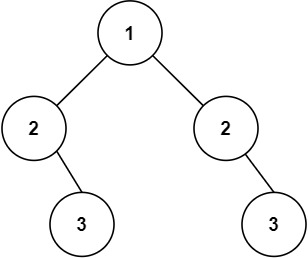
Input: root = [1,2,2,null,3,null,3] Output: false
Constraints:
- The number of nodes in the tree is in the range
[1, 1000]
. -100 <= Node.val <= 100
Follow up: Could you solve it both recursively and iteratively?
Solution(Python)¶
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, val=0, left=None, right=None):
# self.val = val
# self.left = left
# self.right = right
class Solution:
def isSymmetric(self, root: Optional[TreeNode]) -> bool:
return self.iterative(root)
# Time Complexity: O(n)
# Space Complexity: O(h)
def recursive(self, root1, root2=None):
if root1 is None and root2 is None:
return True
if root1 is not None and root2 is not None:
if root1.val == root2.val:
return self.recursive(root1.left, root2.right) and self.recursive(
root1.right, root2.left
)
return False
# Time Complexity: O(n)
# Space Complexity: O(n)
def iterative(self, root):
q = deque([])
q.append(root)
q.append(root)
while q:
original_node = q.popleft()
mirrored_node = q.popleft()
if original_node.val != mirrored_node.val:
return False
if original_node.left and mirrored_node.right:
q.append(original_node.left)
q.append(mirrored_node.right)
elif original_node.left or mirrored_node.right:
return False
if original_node.right and mirrored_node.left:
q.append(original_node.right)
q.append(mirrored_node.left)
elif original_node.right or mirrored_node.left:
return False
return True