1305-all-elements-in-two-binary-search-trees¶
Try it on leetcode
Description¶
Given two binary search trees root1
and root2
, return a list containing all the integers from both trees sorted in ascending order.
Example 1:
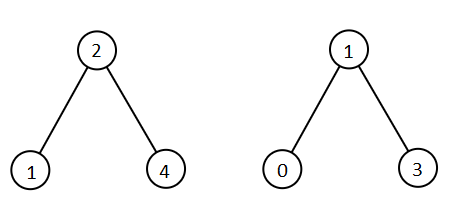
Input: root1 = [2,1,4], root2 = [1,0,3] Output: [0,1,1,2,3,4]
Example 2:
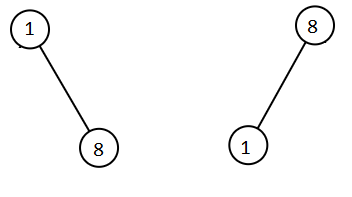
Input: root1 = [1,null,8], root2 = [8,1] Output: [1,1,8,8]
Constraints:
- The number of nodes in each tree is in the range
[0, 5000]
. -105 <= Node.val <= 105
Solution(Python)¶
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, val=0, left=None, right=None):
# self.val = val
# self.left = left
# self.right = right
class Solution:
def inorder(self, root, lst):
if not root:
return
self.inorder(root.left, lst)
lst.append(root.val)
self.inorder(root.right, lst)
def getAllElements(self, root1: TreeNode, root2: TreeNode) -> List[int]:
return self.Linear(root1, root2)
"""
Time Complexity: O((m+n)*log(m+n))
Space Complexity: O(max(m,n))
"""
def bruteforce(self, root1: TreeNode, root2: TreeNode) -> List[int]:
res = []
self.inorder(root1, res)
self.inorder(root2, res)
res.sort()
return res
"""
Time Complexity: O(m+n)
Space Complexity: O(m+n)
"""
def Linear(self, root1: TreeNode, root2: TreeNode) -> List[int]:
lst1, lst2 = [], []
self.inorder(root1, lst1)
self.inorder(root2, lst2)
res = []
i, j = 0, 0
while i < len(lst1) and j < len(lst2):
if lst1[i] < lst2[j]:
res.append(lst1[i])
i += 1
else:
res.append(lst2[j])
j += 1
while i < len(lst1):
res.append(lst1[i])
i += 1
while j < len(lst2):
res.append(lst2[j])
j += 1
return res