230-kth-smallest-element-in-a-bst¶
Try it on leetcode
Description¶
Given the root
of a binary search tree, and an integer k
, return the kth
smallest value (1-indexed) of all the values of the nodes in the tree.
Example 1:
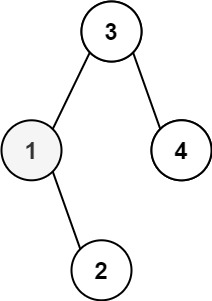
Input: root = [3,1,4,null,2], k = 1 Output: 1
Example 2:
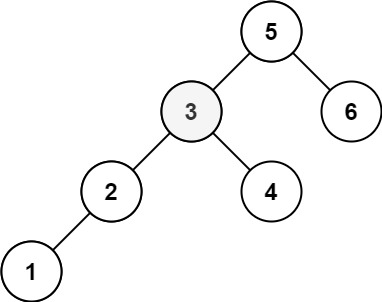
Input: root = [5,3,6,2,4,null,null,1], k = 3 Output: 3
Constraints:
- The number of nodes in the tree is
n
. 1 <= k <= n <= 104
0 <= Node.val <= 104
Follow up: If the BST is modified often (i.e., we can do insert and delete operations) and you need to find the kth smallest frequently, how would you optimize?
Solution(Python)¶
class Solution:
def kthSmallest(self, root, k):
"""
:type root: TreeNode
:type k: int
:rtype: int
"""
cur = root
while cur:
if cur.left is None:
k -= 1
if k == 0:
return cur.val
cur = cur.right
else:
pre = cur.left
while pre.right and pre.right is not cur:
pre = pre.right
if pre.right is None:
pre.right = cur
cur = cur.left
else:
pre.right = None
k -= 1
if k == 0:
return cur.val
cur = cur.right