51-n-queens¶
Try it on leetcode
Description¶
The n-queens puzzle is the problem of placing n
queens on an n x n
chessboard such that no two queens attack each other.
Given an integer n
, return all distinct solutions to the n-queens puzzle. You may return the answer in any order.
Each solution contains a distinct board configuration of the n-queens' placement, where 'Q'
and '.'
both indicate a queen and an empty space, respectively.
Example 1:
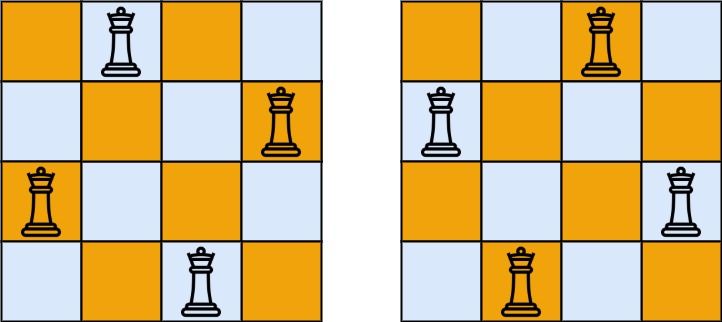
Input: n = 4 Output: [[".Q..","...Q","Q...","..Q."],["..Q.","Q...","...Q",".Q.."]] Explanation: There exist two distinct solutions to the 4-queens puzzle as shown above
Example 2:
Input: n = 1 Output: [["Q"]]
Constraints:
1 <= n <= 9
Solution(Python)¶
class Solution:
def solveNQueens(self, n: int) -> List[List[str]]:
res = []
for solution in self.queens(n, 0, [], [], []):
board = [
"".join("Q" if i == j else "." for i in range(len(solution)))
for j in solution
]
res.append(board)
return res
def queens(self, n, i, a, b, c):
if i < n:
for j in range(n):
if j not in a and i + j not in b and i - j not in c:
yield from self.queens(n, i + 1, a + [j], b + [i + j], c + [i - j])
else:
yield a