63-unique-paths-ii¶
Try it on leetcode
Description¶
You are given an m x n
integer array grid
. There is a robot initially located at the top-left corner (i.e., grid[0][0]
). The robot tries to move to the bottom-right corner (i.e., grid[m-1][n-1]
). The robot can only move either down or right at any point in time.
An obstacle and space are marked as 1
or 0
respectively in grid
. A path that the robot takes cannot include any square that is an obstacle.
Return the number of possible unique paths that the robot can take to reach the bottom-right corner.
The testcases are generated so that the answer will be less than or equal to 2 * 109
.
Example 1:
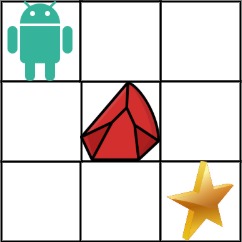
Input: obstacleGrid = [[0,0,0],[0,1,0],[0,0,0]] Output: 2 Explanation: There is one obstacle in the middle of the 3x3 grid above. There are two ways to reach the bottom-right corner: 1. Right -> Right -> Down -> Down 2. Down -> Down -> Right -> Right
Example 2:
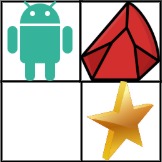
Input: obstacleGrid = [[0,1],[0,0]] Output: 1
Constraints:
m == obstacleGrid.length
n == obstacleGrid[i].length
1 <= m, n <= 100
obstacleGrid[i][j]
is0
or1
.
Solution(Python)¶
class Solution(object):
def uniquePathsWithObstacles(self, obstacleGrid):
"""
:type obstacleGrid: List[List[int]]
:rtype: int
"""
self.obstacleGrid = obstacleGrid
return self.topdown(0, 0)
def inplacedp(self, obstacleGrid):
m = len(obstacleGrid)
n = len(obstacleGrid[0])
# If the starting cell has an obstacle, then simply return as there would be
# no paths to the destination.
if obstacleGrid[0][0] == 1:
return 0
# Number of ways of reaching the starting cell = 1.
obstacleGrid[0][0] = 1
# Filling the values for the first column
for i in range(1, m):
obstacleGrid[i][0] = int(
obstacleGrid[i][0] == 0 and obstacleGrid[i - 1][0] == 1
)
# Filling the values for the first row
for j in range(1, n):
obstacleGrid[0][j] = int(
obstacleGrid[0][j] == 0 and obstacleGrid[0][j - 1] == 1
)
# Starting from cell(1,1) fill up the values
# No. of ways of reaching cell[i][j] = cell[i - 1][j] + cell[i][j - 1]
# i.e. From above and left.
for i in range(1, m):
for j in range(1, n):
if obstacleGrid[i][j] == 0:
obstacleGrid[i][j] = obstacleGrid[i - 1][j] + \
obstacleGrid[i][j - 1]
else:
obstacleGrid[i][j] = 0
# Return value stored in rightmost bottommost cell. That is the destination.
return obstacleGrid[m - 1][n - 1]
def dfs(self, obstacleGrid):
m, n = len(obstacleGrid), len(obstacleGrid[0])
def recur(i, j):
if i == m - 1 and j == n - 1:
return 1
if i >= m or j >= n or obstacleGrid[i][j]:
return 0
return recur(i + 1, j) + recur(i, j + 1)
return recur(0, 0)
@cache
def topdown(self, i, j):
m, n = len(self.obstacleGrid), len(self.obstacleGrid[0])
if i == m - 1 and j == n - 1 and not self.obstacleGrid[i][j]:
return 1
if i >= m or j >= n or self.obstacleGrid[i][j]:
return 0
return self.topdown(i + 1, j) + self.topdown(i, j + 1)